上一篇說明Langchain的SQL Agent範例如何透過ReAct Prompting實作,而目前LLM其實都已經具有Function Calling的能力,比起要解析LLM回傳的Action和Action Input再另外執行,直接透過Function Calling的效果其實更好。甚至目前的LLM能力已經可以在不強迫產生Thought的推論動作下模型就能順利解決問題。
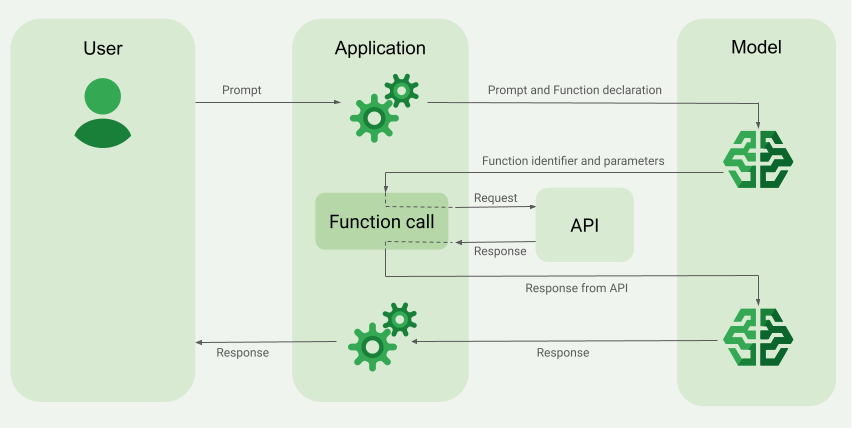
Function Calling可以允許LLM使用外部的API或Function,以克服無法取得最新知識或是外部資料的限制。只要將prompt和可以允許執行的Function提供給LLM,模型就能在處理prompt時選擇能夠處理當下任務的Function並得到回傳資訊。在取得Function所提供的外部回傳資訊後,可以再提供給模型作最後的回答。
下面使用Langchain的SQLDatabase提供執行的Function,與Gemini的Function Calling完成Text-to-SQL任務。
首先建立Chinook資料庫,並使用langchain的SQLDatabase建立資料庫的查詢引擎:
1 | import sqlite3 |
1 | from langchain_community.utilities import SQLDatabase |
Gemini會使用function name, docstring, parameters和parameter type annotations來決定是否要使用特定的函式來回答問題。這裡借用Langchain的SQLDatabase的三個Function並寫好docstring和parameter。
1 | def sql_db_list_tables(): |
接著設定function calling config,這裡設定成ANY會強迫讓模型使用提供的Function,並提供以上三個Function。
1 | from google.generativeai.types import content_types |
以下參考Langchain的prompt內容並擷取prefix當作instruction還有輸入的問題為input。
1 | instruction = """ |
如果使用generate_content會需要手動管理與模型的互動,所以這裡會把將instruction、prompt和tools提供給模型並與模型建立chat session,其中將enable_automatic_function_calling設成True時會允許模型自己呼叫我們所提供的Function。
1 | # Configure Gemini |
最後可以從模型得到正確回答「There are 8 employees.」。
1 | There are 8 employees. |
可以從以下的chat history看到模型會從input的內容開始,先呼叫查詢所有的資料表後,接著選出Employee資料表查詢schema資訊,最後下一段模型生成出來的SQL查詢後回答最終的答案。
1 | for content in chat.history: |
1 | user -> [{'text': '\nHow many employees are there?\n'}] |
參考資料:
Generative-AI function-calling
Gemini cookbook Function_Calling
Gemini cookbook Function_Calling_config